How to Continue an Indented Block in Python
Indentation is a vital feature of readable, maintainable code, but few languages enforce it. Python is one of those few.
If Python determines your code is indented incorrectly, you'll be seeing the "IndentationError" message when you run your code. But how do you fix this, and how do you prevent it in the future?
Why Do You Get the IndentationError in Python?
The "IndentationError: expected an indented block" error is something you're likely to see when you first start using Python, especially if you've come from another programming language.
The specifics of Python's indentation rules are complex, but they boil down to one thing: indent code in blocks. This goes for functions, if clauses, and so on. Here's an example of incorrectly formatted Python code:
fname = "Gaurav"
lname = "Siyal" if fname == "Gaurav" and lname == "Siyal":
print("You're Gaurav")
else:
print("You're somebody else")
When you try to run the above code, you'll get a message like this:
File "tmp.py", line 5
print("You're Gaurav")
^
IndentationError: expected an indented block
Instead, you should add either a tab or a series of spaces at the start of the two lines that represent blocks:
fname = "Gaurav"
lname = "Siyal" if fname == "Gaurav" and lname == "Siyal":
print("You're Gaurav")
else:
print("You're somebody else")
If you indent with spaces, you can actually use any number you like, so long as you're consistent and unambiguous. Most programmers use two, four, or eight spaces.
Common Cases of Correct Indentation
Here are some examples that you can refer to, so you can ensure you're indenting correctly.
If statements
Indent the block that follows an if statement:
if my_name == "Gaurav":
print("My name is Gaurav")
return True
Functions
The body of a function is a block. You should indent this entire block:
def magic_number ():
result = 42
return result print magic_number()
For Loops
As with an if statement, the body of a for loop should be indented one level more than the line starting with the for keyword:
for i in range(10):
print (i)
Make Sure Your Editor Indents Correctly
Most modern text editors support automatic code indentation. If your editor determines that a line of code should be indented, it will add tabs or spaces automatically.
In Spyder, indentation options are available under Tools > Preferences > Source code:
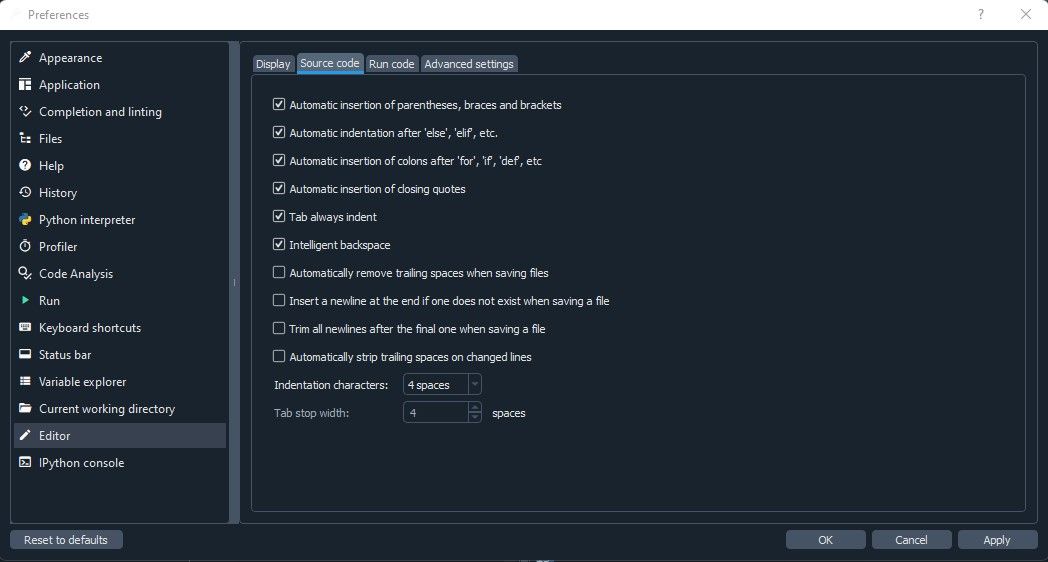
If you're using vim, you can edit your configuration and use the autoindent and related options to configure indentation. For example, here's a common setup:
set autoindent
set expandtab
set tabstop=4
set softtabstop=4
set shiftwidth=4
This will automatically indent using four spaces.
However, no editor can make automatic indentation bulletproof. You'll still need to pay attention to indenting because some cases are ambiguous:
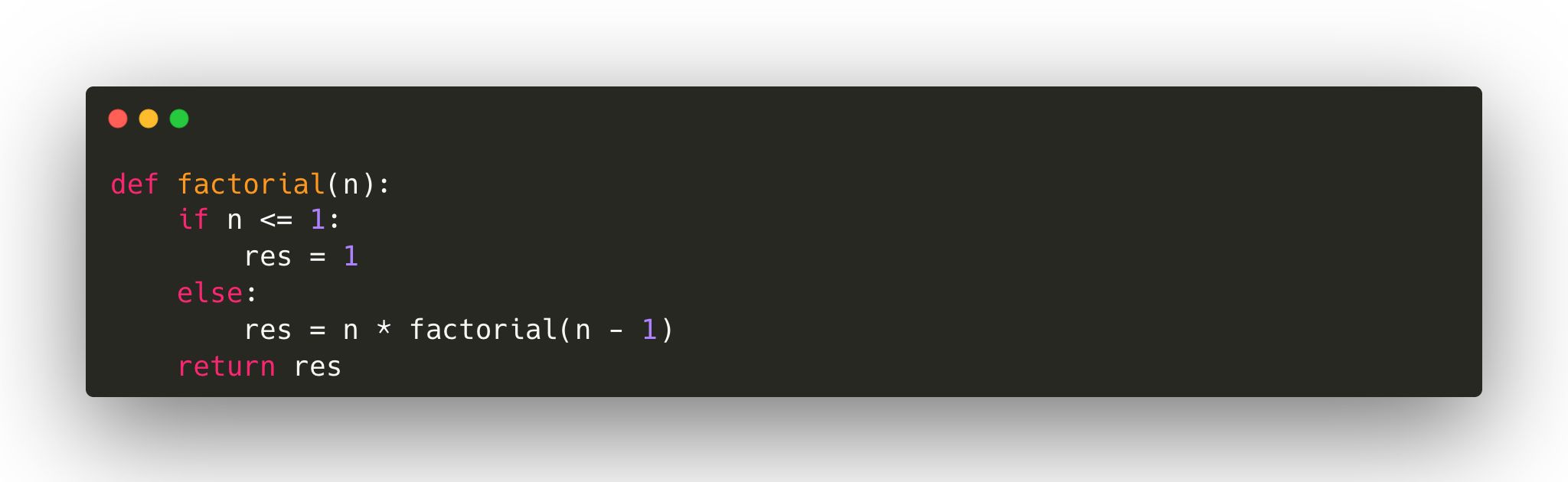
In this example, the final return statement is indented one level in from the function signature on the first line. However, if you position your cursor at the end of the penultimate line and press Enter, one of two things may happen. Your editor could position the cursor:
- Two indent levels in, aligned with "res =..."
- One indent level in, aligned with the "else:"
Your editor cannot distinguish between these two cases: you may want to add more code in the if/else block, or you may not.
Handling Python's 'Expected an Indented Block' Error
Errors are an everyday occurrence in Python, just as in any other programming language. Python's strict rules about indentation may add a new kind of error to think about, but they are useful. Properly indented code is more readable and consistent across teams.
The indentation error is not the only one you'll have to deal with. It helps to be familiar with common Python errors so you know how to debug them and what to do to fix them.
Source: https://www.makeuseof.com/python-indentation-error-expected-block-error-fix/
0 Response to "How to Continue an Indented Block in Python"
Post a Comment